Let's say you need to create a list of "services" or "available training courses" on your website/app. SonicJs is well suited for this task because the core module framework provides a strong collection of tools to allow you to build your own custom content type, manage the CRUD for the new content type via the backend UI, generate a view to display your list and place that view on a page(s).
Warning - When creating new modules, run the app with "npm start" instead of "npm run dev" (which uses Nodemon) in order to prevent the app from restarting while the new module files are being generated.
In order to demonstrate how to generate a listing module, we'll create a list of "services" and place it on a page on our site. Let's walk-thru each step.
To get started, let's head over to the modules page in the back end:
- http://localhost:3018/admin/modules
- Next, click "New Module"
- Enter "Services" into the module title field
- Click "Create Module"
Head back to your module list page and make sure that the new module is now listed:
- http://localhost:3018/admin/modules
- Click on "Services"
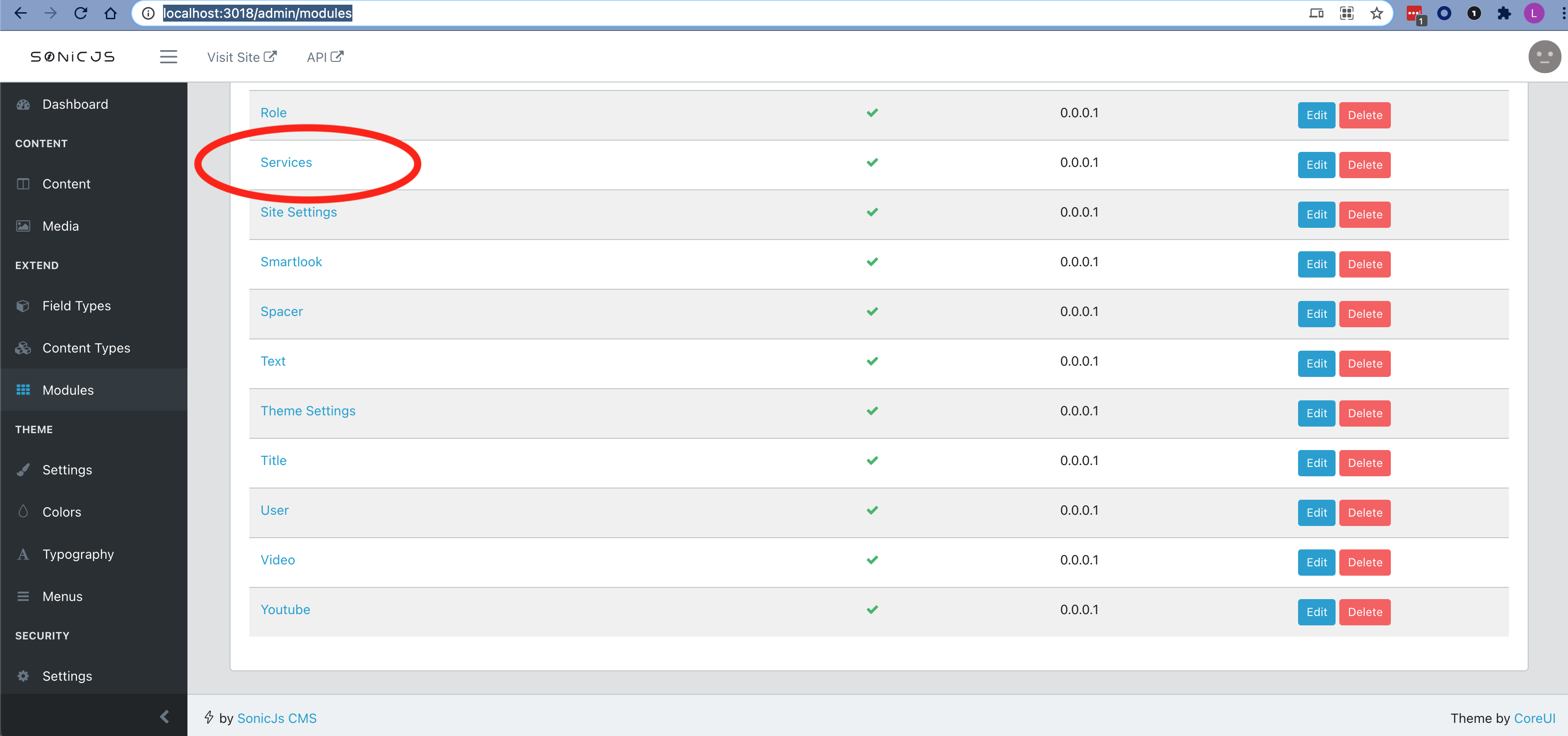
Head back to your module list page and make sure that the new module is now listed:
- http://localhost:3018/admin/modules
- Click on "Services"
- Click "Edit" for the Services content type
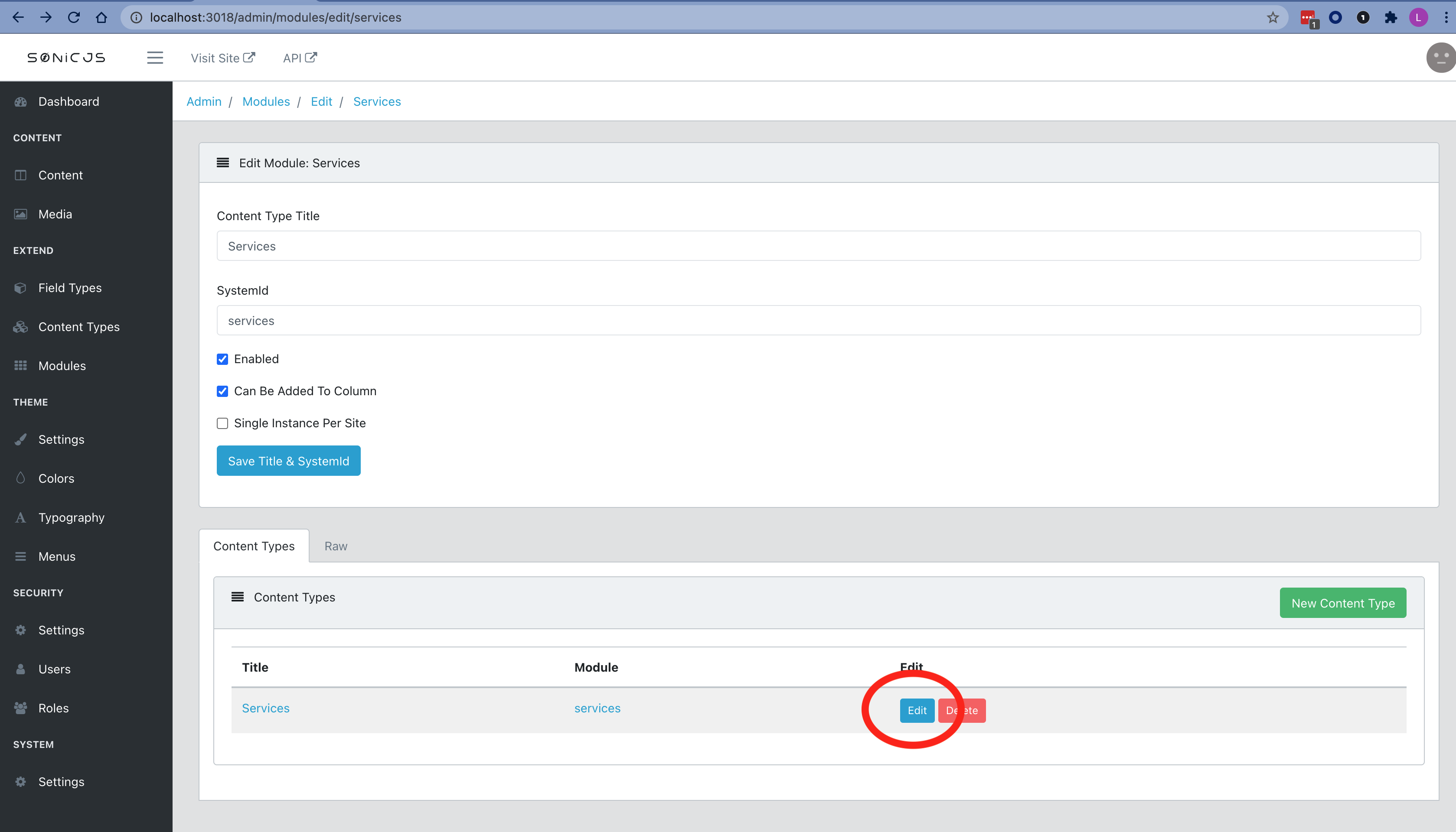
Now its time to drag and drop the fields needed for our "Services" content type. Let's add a textbox for "title" and another textarea for "description". Do this by drag and dropping a "Text Field" from the left field types pain onto the main form canvas:
Let's now switch gears for a minute and generate some content to work with. To do this:
- Visit the content page: http://localhost:3018/admin/content
- Click "New Content"
- Click "Services"
- Add a Title and Description and click Submit
- Repeat this process 1-2 more times to create another Services content instance.
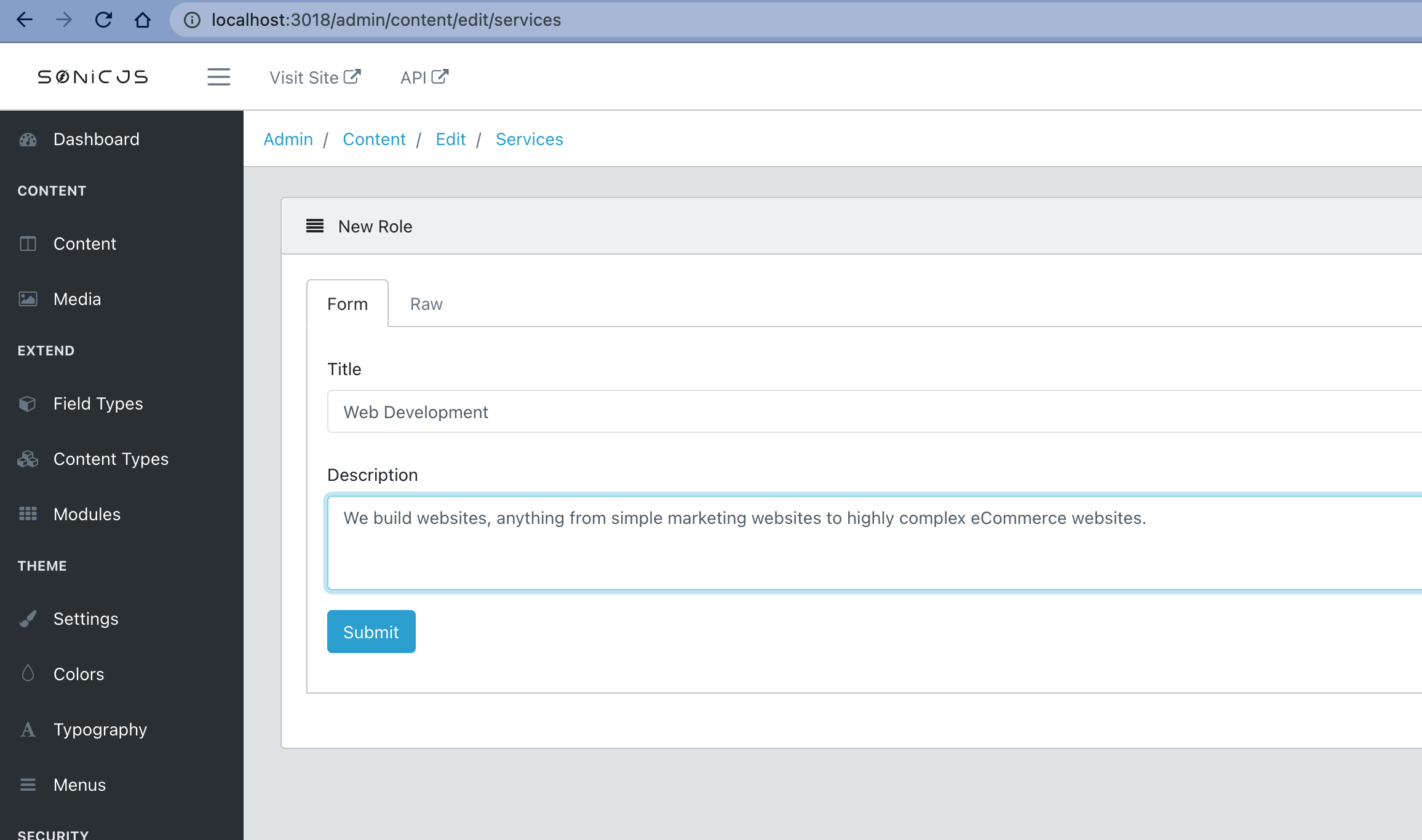
Next let's modify our middle tier code to pass a list of Services to our view.
Open the Service module service file located here:
[sonicjs root path]/server/modules/services/services/services-main-service.js
var dataService = require('../../../services/data.service');
var emitterService = require('../../../services/emitter.service');
var globalService = require('../../../services/global.service');
module.exports = servicesMainService = {
startup: async function () {
// This is boilerplate for proccessing the module inside of a column
// You will rarely need to alter this
emitterService.on('beginProcessModuleShortCode', async function (options) {
if (options.shortcode.name === 'SERVICES') {
options.moduleName = 'services';
await moduleService.processModuleInColumn(options);
}
});
// We want to get a list of all services
// By default SonicJs will pass in the view settings, so we'll extend the
// view model to include our list of services
emitterService.on("postModuleGetData", async function (options) {
if (options.shortcode.name === "SERVICES") {
await servicesMainService.getServicesData(options);
}
});
},
//get all content of type "services", sort it and send it to the view
getServicesData: async function (options) {
let list = await dataService.getContentByType("services", options.req.sessionID);
list = list.sort((a, b) => (a.title > b.title) ? 1 : -1)
options.viewModel.data.list = list;
},
}
Let's update our view to loop thru our services and display them.
[sonicjs root path]/server/modules/services/services/services-main.hbs
{{#each data.list}}
<div class="mb-6">
<h3>{{this.data.title}}</h3>
<p>{{this.data.description}}</p>
</div>
{{/each}}
One last step - we just need to drop our new module onto a page.
- Create a new page called "Services"
- Add a new section by clicking "Add New Section"
- Select the section by click on "Empty Column"
- In the module drop down list, select Services:
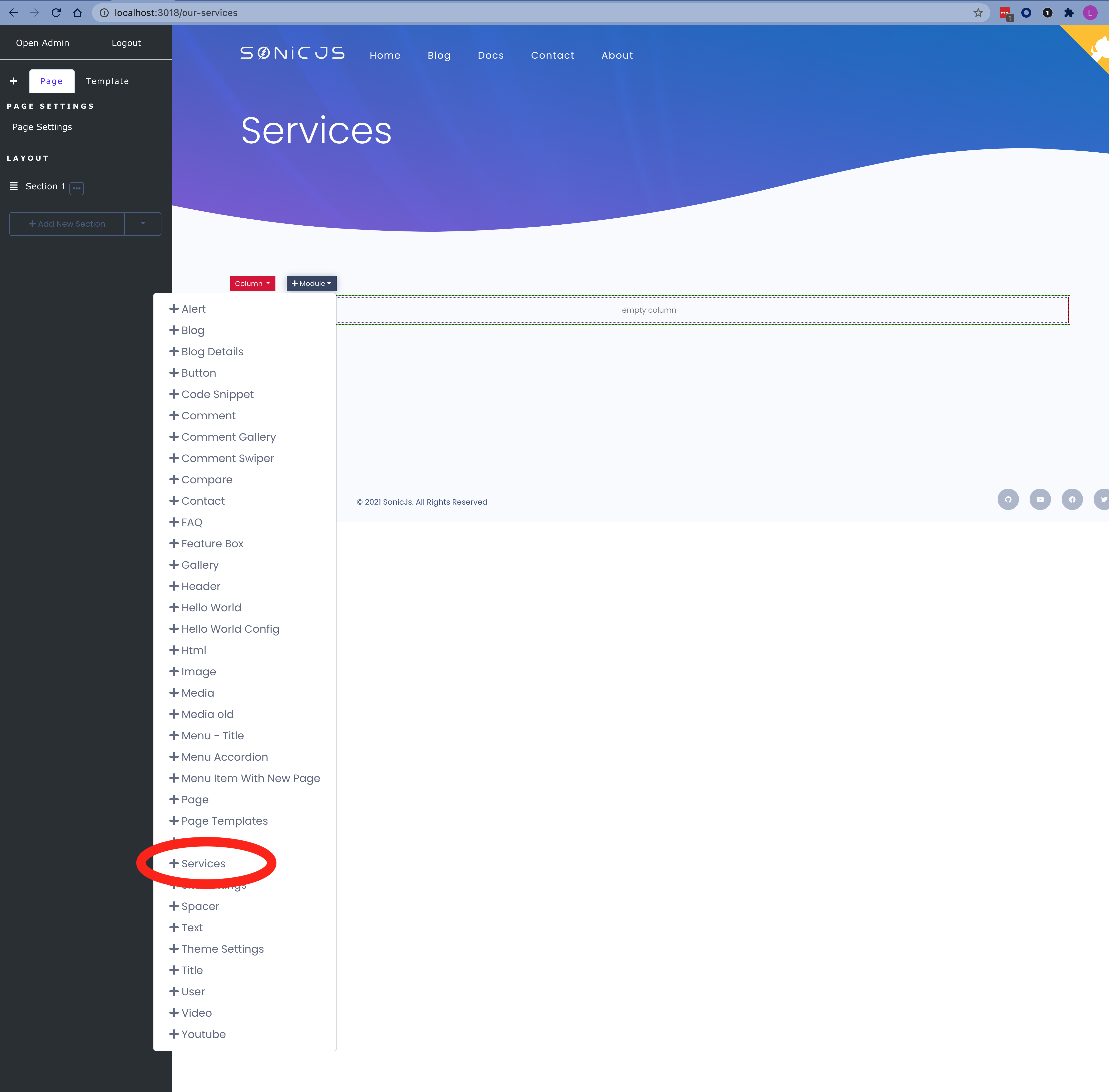
The module setting right sidebar panel will show.
- Leave "Enabled" clicked
- Click "Submit"
You should now see our list of services on the page:
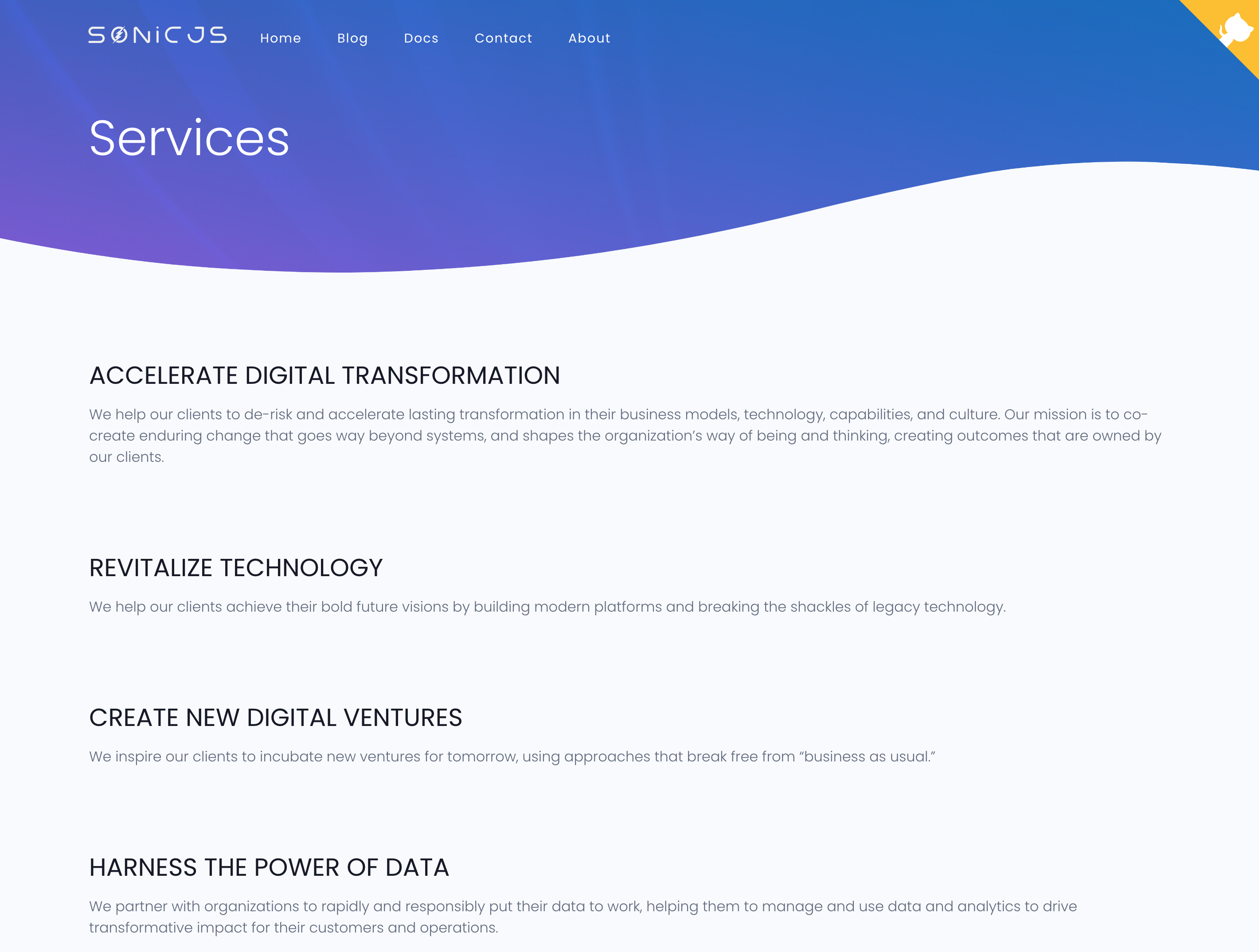
That's it. You have successfully built a listing module. The management of each service record is 100% managed by the back-end with no need to code out any admin pages.
Bonus Steps if you are up for a challenge and want to further explore the awesomeness of SonicJs module development:
- Add a new property for your Service content type, then add that property to the output of the view.
- Add a numeric "sort" field to your Service content type, and sort based on that value
- Add an image for each Service. You can explore how this is done in the blog module for a sample.
- Add validations to the title and description fields by setting them as required
- Change the description field to a WYSIWYG instead of a plain text area. You can explore how this is done in the blog module for a sample.
- Expand on the "Services Settings" content type and use a new setting property in your services-main-service.js or service-main.hbs file.